This post is completed by 1 user
|
Add to List |
190. Swap Nodes in pairs in a Linked List by changing links
Objective: Given a linked list write an algorithm to swap nodes in pairs by changing links.
Earlier we have seen "Swap Every Kth node in a Linked List", where we have seen how to swap nodes by actually swapping the nodes In this article we will see how to swap nodes by changing the links.
Example:
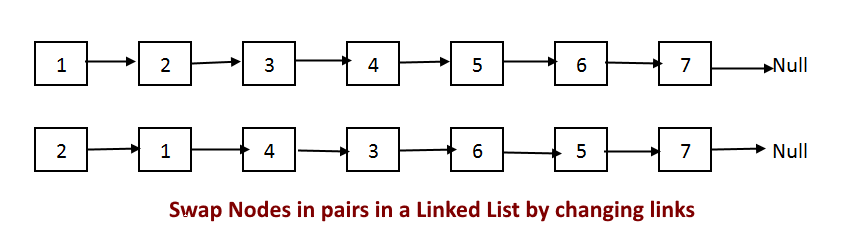
Approach:
Iterative Method:
- Take the 4 pointers ptrOne, ptrOne_prev, newhead and ptrTwo.
- Make ptrOne = head and ptrTwo = head.next.
- Store the reference of next node of ptrTwo, call it ptrTwoNext.
- Make the ptrOne.next = ptrTwoNext. (we have just swap the ptrOne).
- Make ptrOne_prev.next = ptrTwo; if ptrOne_prev is not null (swapped the ptrTwo).
- Make the ptrTwo as newHead (this step will happen only once).
- Move 2 nodes ahead for next pair wise swap.
- See the code for more understanding.
Recursive Method:
- This approach is easy compared to the iterative approach.
- Change the links for the first two nodes.
- Make the recursive call to the rest of the list.
- See the code for more understanding.
Output:
Swapping Nodes using Iterative method ->2->1->4->3->6->5->7 Swapping again using Recursive method ->1->2->3->4->5->6->7