This post is completed by 6 users
|
Add to List |
58. Generate All String Permutations
Objective: Given a String, print all the permutations of it.
Example:
Input : abc Output: abc acb bac bca cba cab
Approach:
- Take one character at a time and fix it at the first position. (use swap to put every character at the first position)
- make recursive call to rest of the characters.
- use swap to revert the string back to its original form fo next iteration.
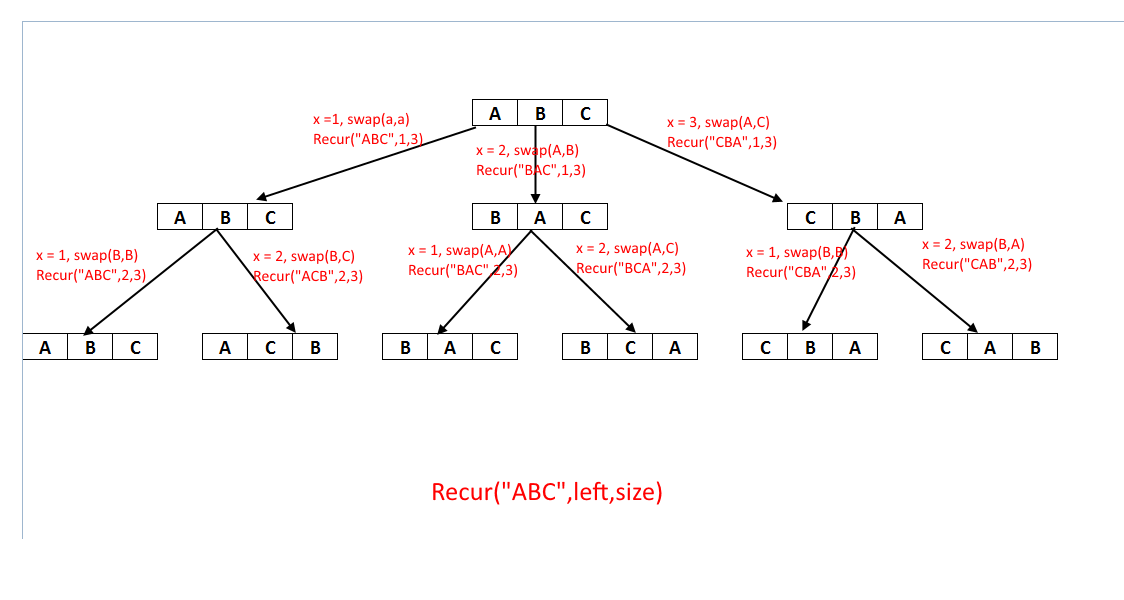
abc acb bac bca cba cab